Introduction
You have landed at the correct place if you want to get your hands on Golang and create a RestAPI using Gin Web Framework. In this, I will be explaining one of my projects were, I created a RestAPI in Golang using Gin Web Framework. Before proceeding with building the project, I would like to mention the prerequisites which you will be required to be prepared with. First, you should have explicit knowledge of concepts like Structs, Slices, Pointers, Routes, Internal Packages, External Packages, and API.
If you are ready with all of the above prerequisites then let’s proceed toward building RestAPI in golang using Gin Framework.
Required Packages
Here, I am mentioning the external packages that I suggest importing before proceeding with writing your code. Since we will be using Gin Web Framework, you need to import the Gin package. The link for Gin Framework is “github.com/gin-gonic/gin”. Next, since we are using MongoDB Atlas for our database, we need to import the MongoDB Driver for the initialization of the program therefore you need to import Mongo Driver from “go.mongodb.org/mongo-driver”. These were some basic external packages that you need to import before proceeding with writing your code.
Usage of Gin Framework
Before proceeding with writing the code, let me give you some brief regarding Gin Web Framework. What is Gin Framework? let me answer that with an example. Basically, In a web application, the client submits an HTTP request, and the server replies by returning the requested information. Any response is possible, including XML, JSON, HTML, etc. We use the “net/http” package in our Golang code to facilitate this request and response process, which is a vital component of maintaining the entire system. net/http package has a drawback in that for complicated programs is not that useful that’s where Gin Web Framework has its upper hand and for complex programming, it is very useful. This itself explains how Golang will be the future in the world of tech, therefore, as an organization if you want to hire Golang developers, you won’t let yourself down. Apart from this, the reason behind being Gin widely used and popular is that you need to write shortcodes and the syntax is not complicated as well.
Code Explanation
Before proceeding, let’s create the folders that you will need to structure the program. Therefore, you need to create a main.go file in the main directory and create three folders named models, database, and controllers. In models, you will create userModels.go. In the database folder, you need to create databaseSetup.go. And in the controllers folder, you need to create userControllers.go.

Now, to understand properly I will start with the main.go file. Here, I have mentioned the main function i.e., “func main ()” where I have defined a port that is empty and added a condition that if there is no port is not available to establish the connection then select the default port as 8080. Then, I have defined the router for POST, GET and DELETE as we will create these three RestAPI for our project. (Refer to Image 1)

Now, moving ahead with our models folder. I have imported the package go.mongodb.org/mongo-driver/bson/primitive. This package contains a type called ObjectID which is used to store MongoDB IDs in Go code. Primitive here, take care that every time the ID is generated for a user, it should unique. I have stated a struct that contains fields like ID, Name, Gender, and Age. (Refer to Image 2)

In the next step, I will be setting up my database. Here in the database, I have defined the function DBSet that connects to the MongoDB database. It returns a pointer to the client object. The client object is used for all operations on the database. And mentioned the variable “Client” and below that stated another function “UserData” that returns a collection from the database. It has two parameters: a client and a collection name. In place of the connection string, you need to fetch down the string from MongoDB Atlas and paste it out there to establish a successful connection with your database. (Refer to Image 3)


Finally, you can work on your userController.go file where we have imported the required packages for which you can refer to Image 4. After that, I worked on our three RestAPI’s and for that, I created three functions i.e., CreateUser, DeleteUser, and GetUser. First comes the CreateUser function that creates a user. It takes in the context of the request and binds it to the User model. If there is an error, it will return a bad request status code and abort the request. Otherwise, it will create an ID for the user and insert them into the database. If there is an error inserted into the database, it will return an internal server error status code and abort the request. Otherwise, it will return a success message with a 200 status code. (Refer to Image 5)

Next, you need to create your GetUser Function that reads an id from the URL and searches the database for a user with that id. If no user is found, an error message is returned. Otherwise, it returns the user’s data in JSON format. (Refer to Image 6)

Finally, I have worked on my DeleteUser function. The DeleteUser function is defined as to deletes a user from the database. It takes in an id and uses it to find the user in the database. If it finds the user, it deletes them. If not, it returns an error message. (Refer to Image 7)
This is how you can create your RestAPI in Golang using Gin Web Framework and I hope you can experience that using Gin makes it so much easier for the developer to write the code and develop the whole structure in a neat and tidy way.
I hope this will be helpful in making you understand the concept of RestAPI and how you can build it in Golang. Happy Coding!!
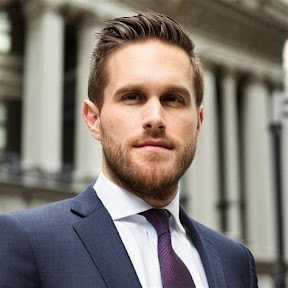
Darren Trumbler is a versatile content writer specializing in B2B technology, marketing strategies, and wellness. With a knack for breaking down complex topics into engaging, easy-to-understand narratives, Darren helps businesses communicate effectively with their audiences.
Over the years, Darren has crafted high-impact content for diverse industries, from tech startups to established enterprises, focusing on thought leadership articles, blog posts, and marketing collateral that drive results. Beyond his professional expertise, he is passionate about wellness and enjoys writing about strategies for achieving balance in work and life.
When he’s not creating compelling content, Darren can be found exploring the latest tech innovations, reading up on marketing trends, or advocating for a healthier lifestyle.