QR codes came into play in place of traditional barcodes. Although these were rarely used, now QR codes are used nearly everywhere for fetching data. Have you wondered what takes a developer of react native app development company to build a QR code scanner app on a mobile device? Let’s check out the steps in detail.
Pre-required learnings
If you have already worked on React Native projects, you may be familiar with the importance of the softwares like Node.js, Virtual device or emulator and Visual studio code. If you have not, firstly need to install all the softwares before getting started with this current project. If you are new to this React Native app development journey, check this article to get a guided step.
Secondly, you have to get a fair knowledge of building a simple React Native app. Get your concept clear from this article.
Use of third-party React Native library
For building a QR code scanner, I have installed two third-party libraries from the npm site.
They are react-native-qrcode-scanner and react-native-camera. A QR code scanner will require a camera component which we will import from react-native-camera. On the other hand, you need to get the native QRCodeScanner from react-native-qrcode-scanner. For both the React Native component, you have to import it from the respective third-party npm package. Most importantly you have to consider the compatibility of the package and the React Native version you are using.
The popularity of the React Native framework over the years has been possible due to its open-source criteria. You will find gigantic support from the React Native community. The availability of third-party plugins for the React Native project is because of the contributions from the entire community. This is one of the benefits using which the React Native experts and react native app development companies create high utility mobile apps.
Detailed understanding of the code syntax
import {StyleSheet, Text, View,TouchableOpacity, Linking } from ‘react-native’
import React from ‘react’
import QRCodeScanner from ‘react-native-qrcode-scanner’;
import { RNCamera } from ‘react-native-camera’;
You will require some React Native components to execute the task. However, for this you have to import the component from the related package and add it in the first few lines. As you can see, I used Text, View, TouchableOpacity, Linking and styleSheet from the ‘react-native’ package. It imported QRCodeScaner and RNCamera from react-native-qrcode-scanner and react-native-camera respectively.
export default function App() {
const onSuccess = e => {
Linking.openURL(e.data)
.catch(err =>
console.error(‘An error occurred’, err)
);
console.log(e.data)
};
A constant variable onSuccess is introduced. Linking component provides a general interface compatible with outgoing and incoming app links. Here, the syntax opens a web link in the browser after you scan the given QR code with the scanner. console.log() and console.error() are used for logging e.data and err respectively. You can also use console.log() for printing the error. However, for better clarity, I have followed this approach.
return (
<QRCodeScanner
onRead={onSuccess}
showMarker={true}
reactivate={true}
This code snippet have props such as onRead, reactivate and showMarker. I have used these props as arguments to the QRCodeScanner. The argument onRead is given a onSuccess value. It is a callback property which will open the URL linked with the QR codes in the net browser. showMarker is true since I wanted to show a marker on the screen surrounding the QR code when detected. Lastly, setting reactivate as true will let users scan multiple codes without any hassle. This implies the scanner will be reactivated to scan another code when the previous code is scanned successfully.
flashMode={RNCamera.Constants.FlashMode.auto}
QRCodeScanner={true}
Here, both flashMode and QRCodeSacnner are considered as arguments. With flashMode prop, I have set the mode of the flash as auto.
topContent={
<Text style={styles.centerText}>
Go to <Text style={styles.textBold}>wikipedia.org/wiki/QR_code</Text>{‘ ‘}
on your computer and scan the QR code.
</Text>
}
bottomContent={
<TouchableOpacity style={styles.buttonTouchable}>
<Text style={styles.buttonText}>OK. Got it!</Text>
</TouchableOpacity>
}
/>
)
}
The syntax specifies what the topContent and bottomContent holds. The content at the top has a text element “Go to wikipedia.org/wiki/QR_code on your computer and scan the QR code” where the text element “wikipedia.org/wiki/QR_code” is bold. The content at the bottom has a touchable button with a text “OK. Got it!”
const styles = StyleSheet.create({
centerText: {
flex: 1,
fontSize: 18,
padding: 32,
color: ‘#777’
},
textBold: {
fontWeight: ‘500’,
color: ‘#000’
},
buttonText: {
fontSize: 21,
color: ‘rgb(0,122,255)’
},
buttonTouchable: {
padding: 16
}
});
Here, you will learn how you can use the StyleSheet for different content (centerText, textBold, buttonText and buttonTouchable). The above given are the parameters (padding, color, fontSize and fontWeight) I have used to design the contents or you can say elements.
Running the virtual device or emulator
Open a terminal or the command prompt. Run two commands: npm install and npx react-native run-android. npm install will install all the dependencies you may need to run the app while npx react-native run-android will start the emulator.
Once the emulator starts, you will see a screen given below.
Give permissions as it asks for. Finally you will start the QR code scanner on your emulator. Use the mouse and the keyboard keyW, A, S, D, Q, and E for moving the camera.
Summary
The process of building QR code scanner may be difficult but once you get your hands on the code editor and and the codebase, it will be exciting. Also, a fair understanding about the React Native third-party plugins and React Native component will also help you in this journey.
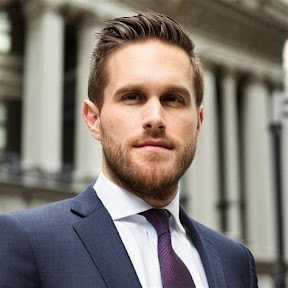
Darren Trumbler is a versatile content writer specializing in B2B technology, marketing strategies, and wellness. With a knack for breaking down complex topics into engaging, easy-to-understand narratives, Darren helps businesses communicate effectively with their audiences.
Over the years, Darren has crafted high-impact content for diverse industries, from tech startups to established enterprises, focusing on thought leadership articles, blog posts, and marketing collateral that drive results. Beyond his professional expertise, he is passionate about wellness and enjoys writing about strategies for achieving balance in work and life.
When he’s not creating compelling content, Darren can be found exploring the latest tech innovations, reading up on marketing trends, or advocating for a healthier lifestyle.